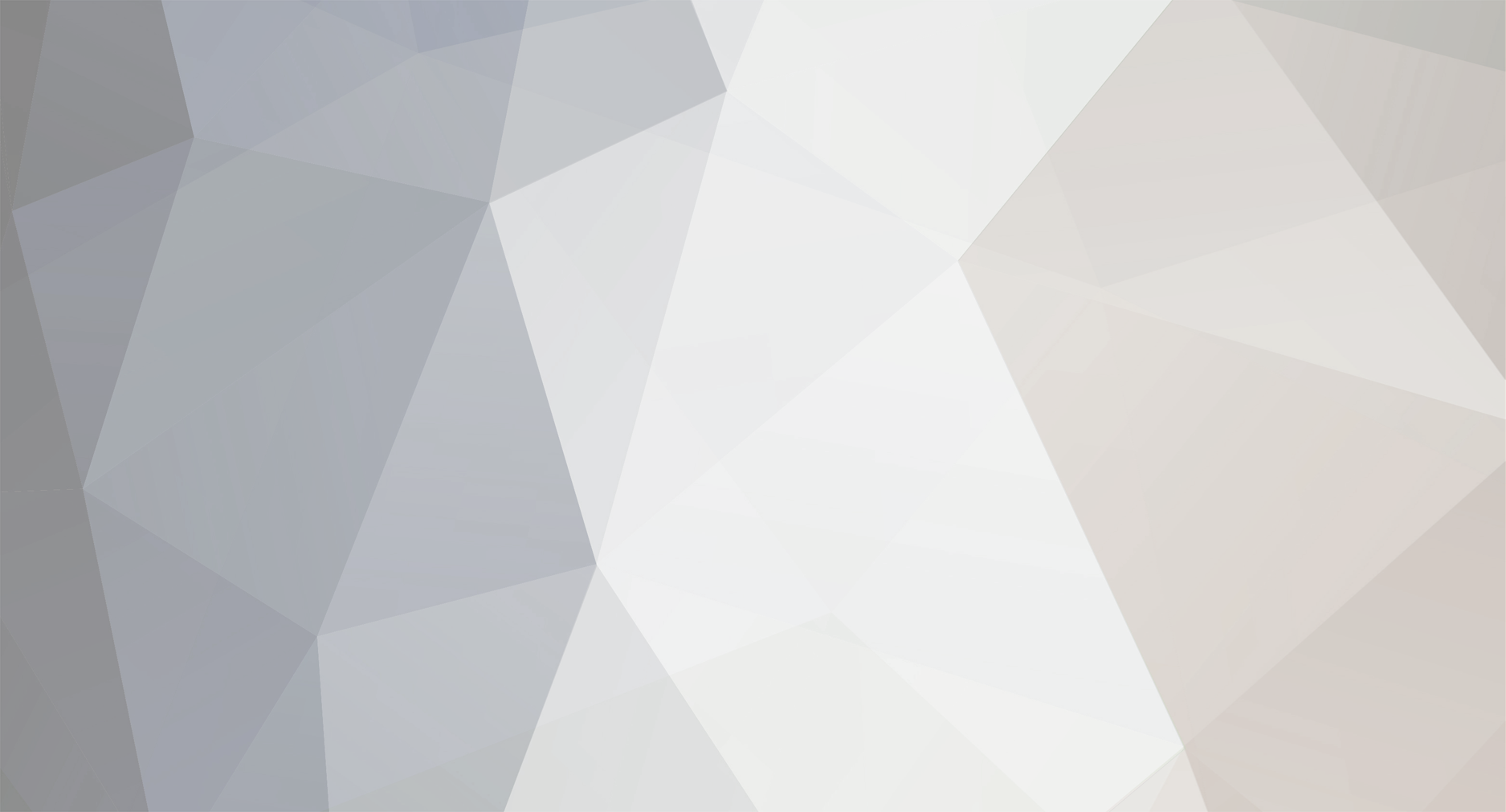
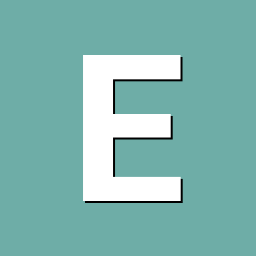
Ed van den Enden
Members-
Posts
15 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
I will leave it for what it is for now. I will move back to RPi zero 2W, solder an antenna, and I'm good to go. Would have loved to go to the BPI m2z and later to the BPI m4z, but for now not an option. By the way, with the latest Armbian upgrade an hour ago, my USB camera is also not working anymore. Thank you very much for the support. Kind regards, Ed👏
-
Nop, that didn't work had also both i2c0 and i2c1 activated root@clearview:~# i2cdetect -y 0 0 1 2 3 4 5 6 7 8 9 a b c d e f 00: -- -- -- -- -- -- -- -- 10: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 20: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 30: 30 -- 32 33 34 35 36 -- -- -- -- -- -- -- -- -- 40: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 50: 50 51 52 53 54 55 56 57 58 59 5a 5b 5c 5d 5e 5f 60: -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- -- 70: -- -- -- -- -- -- -- -- root@clearview:~# i2cdetect -y 1 Error: Could not open file `/dev/i2c-1' or `/dev/i2c/1': No such file or directory root@clearview:~# ls /dev/i2c-* /dev/i2c-0
-
The below output with the RTC module taken off the BPI M2Z Further below I saw that there were some pin errors. (most probably nothing to do with this topic) https://paste.armbian.com/axoduwanuq [ 1.020506] usbcore: registered new interface driver usb-storage [ 1.022284] sun6i-rtc 1f00000.rtc: registered as rtc0 [ 1.022371] sun6i-rtc 1f00000.rtc: setting system clock to 1970-01-01T00:00:04 UTC (4) [ 1.023147] i2c_dev: i2c /dev entries driver [ 1.025025] sunxi-wdt 1c20ca0.watchdog: Watchdog enabled (timeout=16 sec, nowayout=0) [ 7.721526] sun8i-h3-pinctrl 1c20800.pinctrl: pin PD14 already requested by onewire@0; cannot claim for pps@0 [ 7.721577] sun8i-h3-pinctrl 1c20800.pinctrl: pin-110 (pps@0) status -22 [ 7.721597] sun8i-h3-pinctrl 1c20800.pinctrl: could not request pin 110 (PD14) from group PD14 on device 1c20800.pinctrl [ 7.721613] pps-gpio pps@0: Error applying setting, reverse things back
-
When connected to WiFi root@clearview:~# timedatectl status Local time: Wed 2025-04-23 09:56:59 CEST Universal time: Wed 2025-04-23 07:56:59 UTC RTC time: Wed 2025-04-23 07:56:58 Time zone: Europe/Brussels (CEST, +0200) System clock synchronized: no NTP service: active RTC in local TZ: no After power down and disconnected from WiFi root@clearview:~# timedatectl status Local time: Wed 2025-04-23 10:04:43 CEST Universal time: Wed 2025-04-23 08:04:43 UTC RTC time: Wed 1970-01-01 00:02:45 Time zone: Europe/Brussels (CEST, +0200) System clock synchronized: no NTP service: active RTC in local TZ: no
-
Indeed, the hardware clock has been set and checked before power off. 1) date -s "April 22, 2024 11:54:00" 2) hwclock -w 3) hwclock -r 4) battery hwclock 3.6V 5) works flawless with RPi Zero 2W 6) i2cdetect -y 0 shows address 068 Why did you modify the hwclock-set script ? Suggested by many, but without modifying this script, it does not change mallfunction of the hwclock. When connected to WiFi hwclock --verbose tells me following: hwclock from util-linux 2.38.1 System Time: 1745389217.903091 Trying to open: /dev/rtc0 Using the rtc interface to the clock. Last drift adjustment done at 1745317708 seconds after 1969 Last calibration done at 1745317708 seconds after 1969 Hardware clock is on UTC time Assuming hardware clock is kept in UTC time. Waiting for clock tick... ...got clock tick Time read from Hardware Clock: 2025/04/23 06:20:20 Hw clock time : 2025/04/23 06:20:20 = 1745389220 seconds since 1969 Time since last adjustment is 71512 seconds Calculated Hardware Clock drift is 0.000000 seconds 2025-04-23 08:20:18.895813+02:00 After power down and When disconnected from WiFi (my application and so the reason why I need a hwclock to work) hwclock --verbose tells me following: hwclock from util-linux 2.38.1 System Time: 1745389772.399976 Trying to open: /dev/rtc0 Using the rtc interface to the clock. Last drift adjustment done at 1745317708 seconds after 1969 Last calibration done at 1745317708 seconds after 1969 Hardware clock is on UTC time Assuming hardware clock is kept in UTC time. Waiting for clock tick... ...got clock tick Time read from Hardware Clock: 1970/01/01 00:04:02 Hw clock time : 1970/01/01 00:04:02 = 242 seconds since 1969 Time since last adjustment is 1745317466 seconds Calculated Hardware Clock drift is 0.000000 seconds 1970/01/01 01:04:00.760027+01:00
-
I'm unable to make an RTC module to work: RTC module ds3231, BPI M2Z and ARMBIAN Bookworm i2c pins 3 and 5 Driver RTC-ds1307 loaded, because RTC-ds3231 is not available. Why do I need a RTC-module? Often in the field I'm not online but I do need the right time on my observation camera's. My application does not allow me for having a monitor. My system does the recordings by motion detection, and the recordings do need the right time on it. armbian-config i2c0 set Tools installed: apt install python3-smbus i2c-tools i2cdetect -y 0 Detects address 068 and not UU as proof of driver been loaded File "hwclock-set" adapted #if [ -e /run/systemd/system ] ; then # exit 0 #fi Fake hwclock removed sudo apt -y remove fake-hwclock sudo update-rc.d -f fake-hwclock remove armbianEnv.txt overlay and dtoverlay correctly set modules.conf added: rtc-ds1307 Still, after a power down, the hardware clock shows 1970 as the date The same module tested on my RPi Zero 2W, and all is working perfect. If somebody can help me, that would be wonderful.
-
Hardware: Banana pi M2 Zero OS Armbian_community_25.5.0-trunk.185_Bananapim2zero_bookworm_current_6.6.75_minimal pyGPIO - A 'more general' python GPIO library from "chwe". Question: Could the below python script work for a safe "shutdown" of the MBI M2Z? _____________________________________________________________________________ #!/usr/bin/python # shutdown/reboot Banana Pi M2 Zero with pushbutton from pyGPIO.gpio import gpio, connector from subprocess import call from datetime import datetime import time # Initialize GPIO gpio.init() # Pushbutton connected to this GPIO pin shutdownPin = connector.GPIOp11 # Adjust based on your wiring # If button pressed for at least this long, shut down. If less, reboot. shutdownMinSeconds = 3 # Button debounce time in seconds debounceSeconds = 0.1 # Set up the GPIO pin as input with pull-up resistor gpio.setcfg(shutdownPin, gpio.INPUT) gpio.pullup(shutdownPin, gpio.PULLUP) buttonPressedTime = None def buttonStateChanged(pin): global buttonPressedTime if gpio.input(pin) == gpio.LOW: # Button is down (active low) if buttonPressedTime is None: buttonPressedTime = datetime.now() else: # Button is up if buttonPressedTime is not None: elapsed = (datetime.now() - buttonPressedTime).total_seconds() buttonPressedTime = None if elapsed >= shutdownMinSeconds: # Button pressed for more than specified time, shutdown call(['shutdown', '-h', 'now'], shell=False) # Uncomment the following lines if you want to enable reboot functionality # elif elapsed >= debounceSeconds: # # Button pressed for a shorter time, reboot # call(['shutdown', '-r', 'now'], shell=False) # Subscribe to button presses gpio.set_irq(shutdownPin, gpio.BOTH, buttonStateChanged) while True: # Sleep to reduce unnecessary CPU usage time.sleep(5)