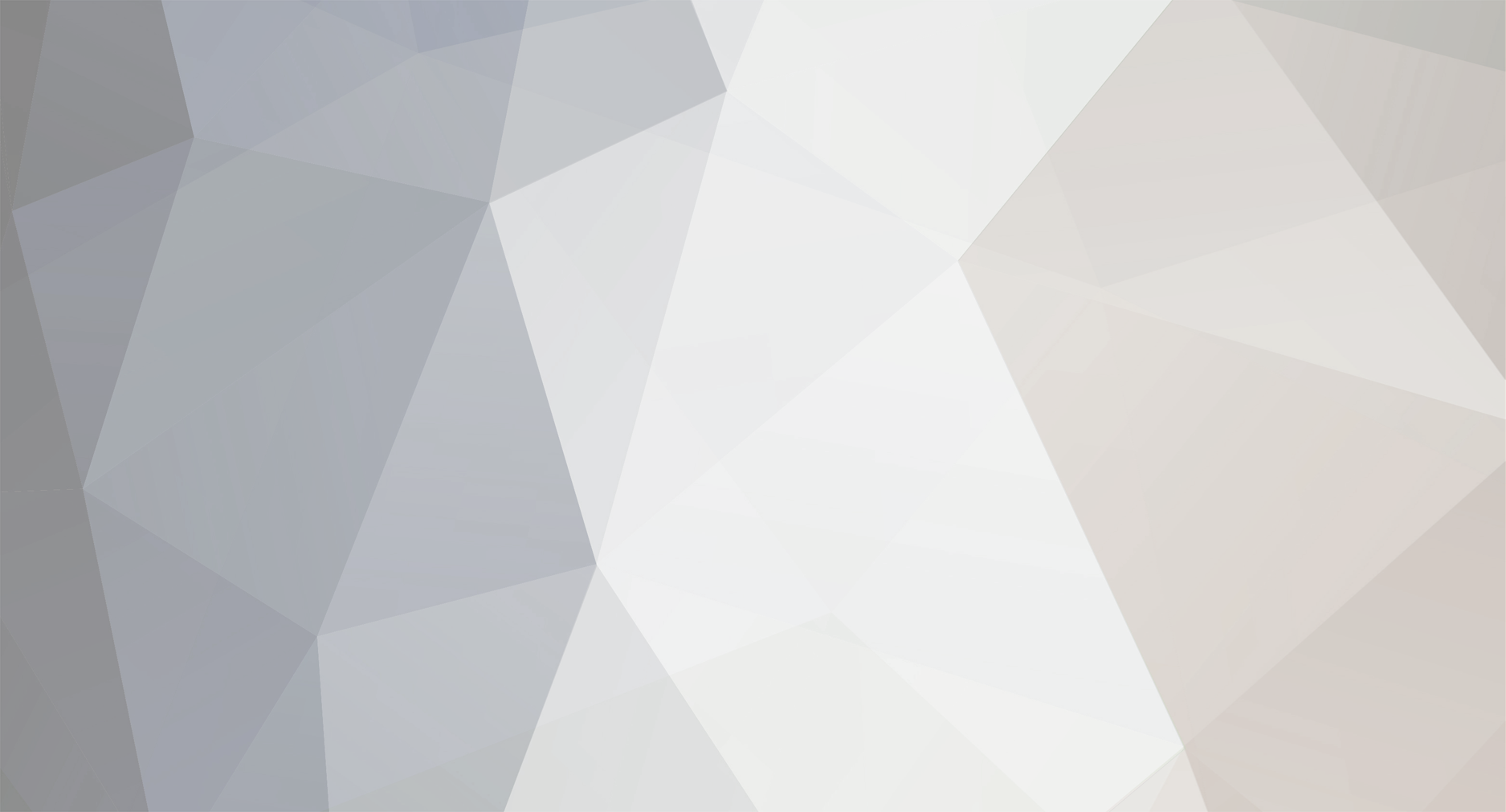
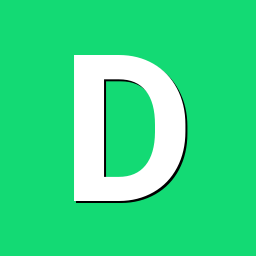
DoubleHP
Members-
Posts
46 -
Joined
-
Last visited
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
-
In case the other website goes down, I will duplicate their original code here. Don't forget to fix your PIN number. My WiringPi lib is probably https://github.com/zhaolei/WiringOP (not 100% certain). /* * dht.c: * read temperature and humidity from DHT11 or DHT22 sensor */ #include <wiringPi.h> #include <stdio.h> #include <stdlib.h> #include <stdint.h> #define MAX_TIMINGS 85 #define DHT_PIN 3 /* GPIO-22 */ int data[5] = { 0, 0, 0, 0, 0 }; void read_dht_data() { uint8_t laststate = HIGH; uint8_t counter = 0; uint8_t j = 0, i; data[0] = data[1] = data[2] = data[3] = data[4] = 0; /* pull pin down for 18 milliseconds */ pinMode( DHT_PIN, OUTPUT ); digitalWrite( DHT_PIN, LOW ); delay( 18 ); /* prepare to read the pin */ pinMode( DHT_PIN, INPUT ); /* detect change and read data */ for ( i = 0; i < MAX_TIMINGS; i++ ) { counter = 0; while ( digitalRead( DHT_PIN ) == laststate ) { counter++; delayMicroseconds( 1 ); if ( counter == 255 ) { break; } } laststate = digitalRead( DHT_PIN ); if ( counter == 255 ) break; /* ignore first 3 transitions */ if ( (i >= 4) && (i % 2 == 0) ) { /* shove each bit into the storage bytes */ data[j / 8] <<= 1; if ( counter > 16 ) data[j / 8] |= 1; j++; } } /* * check we read 40 bits (8bit x 5 ) + verify checksum in the last byte * print it out if data is good */ if ( (j >= 40) && (data[4] == ( (data[0] + data[1] + data[2] + data[3]) & 0xFF) ) ) { float h = (float)((data[0] << 8) + data[1]) / 10; if ( h > 100 ) { h = data[0]; // for DHT11 } float c = (float)(((data[2] & 0x7F) << 8) + data[3]) / 10; if ( c > 125 ) { c = data[2]; // for DHT11 } if ( data[2] & 0x80 ) { c = -c; } float f = c * 1.8f + 32; printf( "Humidity = %.1f %% Temperature = %.1f *C (%.1f *F)\n", h, c, f ); }else { printf( "Data not good, skip\n" ); } } int main( void ) { printf( "Raspberry Pi DHT11/DHT22 temperature/humidity test\n" ); if ( wiringPiSetup() == -1 ) exit( 1 ); while ( 1 ) { read_dht_data(); delay( 2000 ); /* wait 2 seconds before next read */ } return(0); }
-
WORKS FOR ME It was my VERY LAST try before giving up completely: https://www.uugear.com/portfolio/read-dht1122-temperature-humidity-sensor-from-raspberry-pi/ fix the ping to 7 (instead of 3 in the source), compile with cc -Wall dht.c -o dht -lwiringPi -pthread and it does the job: According to other forums, 60% misses is ordinary for this probe. Note that the very first value is completely wrong; I did not read anything about this, but I am not surprised. CO2 probes need 15 pre heat; and this is the very first successfull read of the probe, so some miss-configuration or badly initialised variable are likely. So, on production line, I will take care to ignore the first 3 values after service start/reboot.
-
For example, I see a /lib/modules/./4.13.16-sunxi/kernel/drivers/iio/humidity/dht11.ko , how can I try using this ?
-
Hello. First, I have an LTS board that provides only 3.05 on the 3.3 pins. Is it normal ? Anyway, in case this was the issue, I have built a new voltage regulator, and now supply the DHT22 and pullup resistor with 3.60V My issue is simple: when plugged on normal 1W bus, the DHT22 kills the bus, and makes other devices non working. I have moved all other devices on a new bus, leaving the DHT on a bus I know works fine. Some people mentionned the DHT is not compliant with official 1W libraries, and can not be recognised with normal timings, so, special libs are required. I have removed param_w1_pin=PA06 for the hysical pin 7, and installed new software. I have a very bad experience with python, and I have never been able to make any python lib/project, on any board of any brand; I always get some error at some point. The only non python lib/app for DHT22 I have found is https://github.com/pilkch/climbatize but after heavily patching it (to fix various bugs), the app still seems unable to communicate with the probe. I have checked more than 12 times the sensor is connected to the so called pin 7 (PA06), pin 2 in WiringPi, and changed the physical sensor (paid for a new item). Who can help reading this probe ? I am just running out of ideas. The only issue that bothers me is that I am not physically 100% certain that Climbatize manipulates pin7. I have entered the loop that manipulates some pin, but at some point it's calling wiringPi, and my multimeter always shows 3.6V on the pin; I don't have a scope to check the pin goes down, and applying a LED on a pulledup pin will not produce a perceptible OFF time. I would need to solder an inverted gate to transform the logic, but even then, the light pulses may be too short for my eyes. I really dont think 3.60V could burn the oPi board; and 3.05 could be a huge reason for the DHT22 to refuse to work. I could plug a lab regulated supply, but I think it would be overkill. I do have spare/new/unused boards; but all my LTS boards deliver 3.05. (yes I have some boards left in 2022; I had bought *MANY* spare ones years ago).
-
Hello; on OrangePi Zero LTS I have completely stupid values for CPU temperature. They were all reasonable for pre-LTS boards. I have read somewhere that this is a known bug. A kernel patch is probably available, but even if it's the case,I can not update my kernel. Where can I find the algorythm to compensate the value ? It's easier for me to fix the issue in userland code, rather than updating kernel. How can I distinguish between pre-LTS and LTS boards ? Maybe MAC address for LTS are always in a given range ? I have a legacy board with MAC 12:42:98:f9:c8:XX, and a LTS with 12:42:3a:63:04:XX (unfortunately the LTS has a lower MAC :/ does not seem chronological )
-
Yes, much better: # ls -lha /var/log | grep -e syslog -e message drwxrwxrwx 11 root syslog 660 Oct 19 19:46 . -rwxrwxrwx 1 root root 6.7K Oct 19 20:43 syslog Thanks
-
Armbian 5.25 on OrangePI PC: The gc2035 video camera doesn't work
DoubleHP replied to IgZero's topic in Allwinner sunxi
I also would perform a sleep 1 or sleep 3 between the two. GPIO handling may take some time, on chipset side. If the pins we talk about are power, or ENABLE pins for the client, even longer time may be required (for internal firmware to startup). Before complaining /dev/video0 does not appear, you need to report the output of dmesg, which gives details about what driver found ... or not. Also check 10 times your sunxi line; many tuto may give wrong addresses; and sometimes even for the same board, different libs may use different numbering schemes ... (had the issue on rPi). -
# df -h | grep log armbian-ramlog 50M 5.7M 45M 12% /var/log # ls -lha /var/log | grep -e syslog -e message drwxrwxr-x 11 root syslog 660 Aug 11 22:26 . -rw-r----- 1 root root 0 Aug 11 22:15 syslog # systemctl status syslog ● rsyslog.service - System Logging Service Loaded: loaded (/lib/systemd/system/rsyslog.service; enabled; vendor preset: enabled) Active: active (running) since Sun 2019-08-11 22:25:49 CEST; 9min ago Docs: man:rsyslogd(8) http://www.rsyslog.com/doc/ Main PID: 1042 (rsyslogd) CGroup: /system.slice/rsyslog.service └─1042 /usr/sbin/rsyslogd -n Aug 11 22:25:49 opi-06-app-c13 systemd[1]: Starting System Logging Service... Aug 11 22:25:49 opi-06-app-c13 systemd[1]: Started System Logging Service. # ls -lha /var/log.hdd/ | grep -e syslog -e message drwxrwxr-x 11 root syslog 4.0K Aug 11 22:25 . -rw-r----- 1 root root 0 Aug 11 22:15 syslog -rw-r----- 1 root root 28M Mar 4 06:25 syslog.1 -rw-r----- 1 root root 212K Mar 3 06:25 syslog.2.gz -rw-r----- 1 root root 2.9M Mar 2 06:25 syslog.3.gz -rw-r----- 1 root root 1.9M Mar 1 06:25 syslog.4.gz -rw-r----- 1 root root 791K Feb 28 06:25 syslog.5.gz it probably worked at some point ... forgot what I did to break it ...
-
I have completely reinstalled the system. Beh ... I don't understand the difference. image: Armbian_5.75_Orangepione_Debian_stretch_next_4.19.20.7z BOARD_NAME="Orange Pi One" BOARDFAMILY=sun8i VERSION=5.75 PRETTY_NAME="Debian GNU/Linux 9 (stretch)" NAME="Debian GNU/Linux" VERSION_ID="9" VERSION="9 (stretch)" ID=debian Linux opi55 4.19.20-sunxi #5.75 SMP Sat Feb 9 19:02:47 CET 2019 armv7l GNU/Linux # aptitude install rcconf sqlite3 sed awk bc munin-node netcat bc socat facter xinit xserver-xor g-video-all xserver-xorg-video-fbdev xinit xserver-xorg-video-all xserver-xorg-video-fbdev aterm xterm wmaker eterm xfonts-base xserver-xorg x11-utils x11-xserver-utils xinput-calibrator xinput dillo munin-plugins-extra zip # startx & # export DISPLAY=":0.0" # cvt 800 480 50 # 800x480 49.69 Hz (CVT) hsync: 24.70 kHz; pclk: 24.50 MHz Modeline "800x480_50.00" 24.50 800 824 896 992 480 483 493 497 -hsync +vsync # xrandr --addmode "HDMI-1" 800x480_50.00 # cvt 800 480 60 # 800x480 59.48 Hz (CVT) hsync: 29.74 kHz; pclk: 29.50 MHz Modeline "800x480_60.00" 29.50 800 824 896 992 480 483 493 500 -hsync +vsync # xrandr --newmode "800x480_60.00" 29.50 800 824 896 992 480 483 493 500 -hsync +vsync # xrandr Screen 0: minimum 320 x 200, current 1280 x 720, maximum 8192 x 8192 HDMI-1 connected primary 1280x720+0+0 (normal left inverted right x axis y axis) 697mm x 392mm 1280x720 60.00*+ 50.00 59.94 1920x1080 60.00 50.00 59.94 1920x1080i 60.00 50.00 59.94 1280x1024 75.02 1440x900 74.98 59.90 1024x768 75.03 70.07 60.00 800x600 72.19 75.00 60.32 56.25 720x576 50.00 720x480 60.00 59.94 640x480 75.00 72.81 60.00 59.94 720x400 70.08 800x480_50.00 49.69 800x480_60.00 (0x6d) 29.500MHz -HSync +VSync h: width 800 start 824 end 896 total 992 skew 0 clock 29.74KHz v: height 480 start 483 end 493 total 500 clock 59.48Hz # xrandr --addmode "HDMI-1" 800x480_60.00 # xrandr -s 800x480_50.00 # xrandr --output HDMI-1 --mode 800x480_60.00 Both last commands work ... do not return error. With the 3.5" LCD, in all 3 cases, I have an image (not fitting edges, but that's offtopic). And now my 19" monitor also works, but with a trick: - run xrandr to have an eye at things - unplug old monitor - run xrandr to have an eye at things, and check that less resolutions are listed (the only ones left are the current one, and the two manually added ones) - plug monitor; it may not produce any image - ask xrandr again to show supported resolutions - run xrandr -s to set any resolution, even the current one, and then, an image will appear. 5" LCD also worked immediately. So, the main difference I can see between the two attemps is the image used, and kernel. 3.4 vs 4.19
-
What's the difference between gtf and cvt ? # gtf 800 480 60 # 800x480 @ 60.00 Hz (GTF) hsync: 29.82 kHz; pclk: 29.58 MHz Modeline "800x480_60.00" 29.58 800 816 896 992 480 481 484 497 -HSync +Vsync cvt 800 480 60 # 800x480 59.48 Hz (CVT) hsync: 29.74 kHz; pclk: 29.50 MHz Modeline "800x480_60.00" 29.50 800 824 896 992 480 483 493 500 -hsync +vsync # xrandr --newmode "800x480_50.00" 24.50 800 824 896 992 480 483 493 497 -hsync +vsync xrandr: Failed to get size of gamma for output default root@orangepione:~# xrandr --addmode "default" 800x480_50.00 xrandr: Failed to get size of gamma for output default xrandr: cannot find mode "800x480_50.00" # xrandr xrandr: Failed to get size of gamma for output default Screen 0: minimum 1280 x 720, current 1280 x 720, maximum 1280 x 720 default connected 1280x720+0+0 0mm x 0mm 1280x720 0.00* How to addmode 800x480 ? BOARD_NAME="Orange Pi One" Image: Armbian_5.75_Orangepione_Ubuntu_xenial_default_3.4.113_desktop.7z I have a correct image using a 3.5" HDMI LCD (without using GPIO/SPI at all); but when I plug a 5" or 19", they say "no signal". I am surprised by two facts: - pi unable to probe monitors correctly over HDMI - 5" LCD designed for rPi unable to accept a modline the opi sends (a modline that is 100% acceptable for the 3.5").
-
Mike R9FT, I have huge doubts ... you claim to be able to make things work with Armbian_5.27.170521_Orangepione_Ubuntu_xenial_dev_4.11.1.7z , but you quote "overlays=spi-spidev" which is a kernel v3 specific option, and ignored by kernels v4 ... After adding sun8i-h3-spi-ads7846.dts , my /dev/fb0 goes away. orangepi zero 4.14.18-sunxi user_overlays=myili9431
-
Armbian 5.25 on OrangePI PC: The gc2035 video camera doesn't work
DoubleHP replied to IgZero's topic in Allwinner sunxi
At last, I have found something that works, and produces images. In short, use ubuntu_lxde_desktop_OrangePipc_v0_9_1.img . Long story is here: http://www.orangepi.org/orangepibbsen/forum.php?mod=viewthread&tid=4270&page=1&extra=#pid25253 Edit: I also suceeded with image Armbian_5.75_Orangepione_Ubuntu_xenial_default_3.4.113_desktop.7z -
Armbian 5.25 on OrangePI PC: The gc2035 video camera doesn't work
DoubleHP replied to IgZero's topic in Allwinner sunxi
Notify me. -
Armbian 5.25 on OrangePI PC: The gc2035 video camera doesn't work
DoubleHP replied to IgZero's topic in Allwinner sunxi
In short, there is no hope to get the camera working on 4.x kernels, because they don't have CSI support ATM. I did not get it to work yet, but, first, grab something using 3.4.x kernel. Spent 3 days on it, still stuck. Also, try gc_2035 ... just in case ... or with dash ... not sure.